In this week’s new videos we are diving head first into PhpSpec.
I am fully aware that this is a series that is aimed at beginners, but in my opinion it’s never too early to learn how to test.
Before we go further though, I do want to stress that PhpSpec is a highly opinionated approach to testing, and that opinion may not be one that you agree with. Or, more importantly, enjoy the feeling you get when you use it.
There are a couple of alternatives to PhpSpec that I have used and would recommend:
But this is somewhat misleading, as Codeception uses PhpUnit for unit testing.
Ok, so at this point, if you are someone new to testing, you may be feeling confused. A very valid question here is:
What the heck is unit testing?
My definition of unit testing is testing individual functions / methods.
There’s major benefits to doing this, which I am hoping to explore with you in these next few videos.
Let’s quickly cover unit testing by way of an example. Imagine we have a function that adds two numbers together:
function add($x, $y)
{
return $x + $y;
}
We could manually test this:
echo add(1,2);
And we should expect to see 3.
One of the first things we do when writing code is find a way to output what we’ve done – to check if what we are doing is working. In PHP we can do this in a variety of ways: die , var_dump , echo , print_r , the list goes on.
This is a form of testing.
If all we are testing here is the output of a single function then heck, this is manual unit testing.
The problem is: we generally don’t have just one function. And if we do, that’s a different kind of problem in itself 🙂
Instead, what we will likely do is start using this add function in a variety of situations throughout our code base.
We might manually test those functions as well. In doing so, we indirectly test the other functions that function depends on.
And that’s all good.
Whilst all this logic is fresh in your head – whilst you’re deep into the systems internals – it’s all there as clear as day. But then another problem arises and you get sidetracked.
And when you return to this code even just a short time later, somehow the fog has begun to set in. You’re not quite sure how that method works anymore. You aren’t able to interpret that 70 line method exactly in your head.
At this point our untested code immediately becomes legacy code. At least, that’s my opinion.
We want to start refactoring it, to fix up all the confusion and restore that prior clarity.
But we can’t because the more we meddle, the more stuff breaks. Or may break. Coding is so stressful!!!!
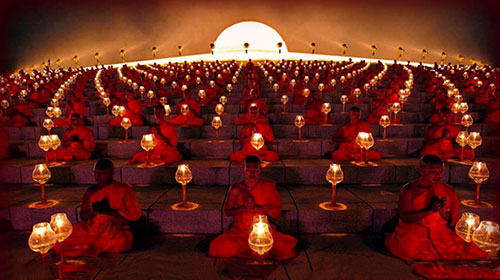
Or, you can swap all this for zen.
You know your code works because the tests pass. Those tests didn’t pass to begin with. You’ve had to write code to prove how your system works. And now when you change your code, if your tests still pass then your system still works exactly as you intended.
And it’s surprising how, when doing this, you will break so many unexpected things when changing seemingly unrelated bits and pieces.
This takes your skill level further. You start to learn about better design as a result.
I’ve found PhpSpec will lead you towards a certain design.
That design is the distilled guidance from very clever people. Why not leverage their knowledge to help your project, but also you – to make your life easier, and less stressful?
Back to our function.
What if we had created our function using unit tests for guidance?
Well, we could take a look at using a unit test suite to write tests for this function.
However, the reality of it is, if you’re working with Symfony, you’re going to be writing code in a certain way.
Why not learn how to write unit tests in an environment just like your real world projects?
I think this is a better way to learn. It’s a little more effort upfront, but you’re learning how it can really and immediately help you become better on the type of code you have to write in your day job.
And so that’s what we are doing in these three videos:
https://codereviewvideos.com/course/let-s-build-a-wallpaper-website-in-symfony-3/video/testing-with-phpspec-to-guide-our-implementation
In case you haven’t been following along, in the past three video’s we’ve been learning how we can use EasyAdminBundle as a quick way to add a really nice UX to our admin area.
We can manage all our existing wallpapers from the back end, but it would be super useful to us if we could handle new wallpaper file uploads from the admin area, too.
EasyAdminBundle comes with a documented way to integrate with VichUploaderBundle.
We could have chosen to go that route, again leveraging the wisdom of the collective.
Instead we are doing some DIY. Our design decisions are not about sharing this code. We’re just exploring some concepts. We want to learn about file uploads, and hopefully improve ourselves as developers a little in the process.
The thing is, handling uploads is code that will be super important to the site and, if it all works, it will be used a lot.
This is the sort of problem that if you don’t handle up front, you’re going to be getting frantic calls from bosses and clients to fix as a matter of up-most urgency :/
No one wants to deal with that. Not you. Not your boss. Not the client.
So, when I hit on code like that, I reach for the unit tests.
https://codereviewvideos.com/course/let-s-build-a-wallpaper-website-in-symfony-3/video/using-phpspec-to-test-our-filemover
In the previous video we covered a little of how the design decisions came about, and started our test routine.
Now we’re going to write some specifications of how we expect this system to behave, if it is performing as expected.
🙂
This is the insurance policy that allows us to refactor our confusing code later on.
If the implementation we write now makes the tests pass, we can know for sure if our code changes don’t lead to failing tests, we have altered the logic without altering the outcome.
If your project survives, this makes it’s lifetime more enjoyable for you as a developer.
You’ll inevitably get to work on more features, not constant and stressful bug fixing.
https://codereviewvideos.com/course/let-s-build-a-wallpaper-website-in-symfony-3/video/symfony-dependency-testing-with-phpspec
Finally we get into how all this integrates with Symfony.
Understand these three pieces and the process essentially repeats over and over for whatever you want or need to test.
The truth about testing is that it’s a pain to get started.
But once you have tested one thing, you can use that one single thing as a reference and it becomes A LOT easier to add tests to an already tested system.
Ok, so that’s why I love testing.
Hopefully if you can invest just 15 minutes into your PHP skills this weekend, it will be these minutes you choose 🙂
I’d love to hear your thoughts on this series so far.
I’m also under way with the big back end refresh once again. I’m going to be deploying this new site as the topic of the upcoming Docker series, which I think is quite a cool way to cover it. It will at least show you how it all works behinds the scenes, if nothing else.
If you haven’t done so recently, why not check out what else is on the site?
Thanks for reading, and have a great weekend,
Chris