I’ve been trying – in vain – to build the front end for CodeReviewVideos. The issue I have been hitting upon is as follows:
ERROR in app.a2e9a6b7afa471d94d2b.js from UglifyJs Unexpected token: name (DropIn) [./node_modules/braintree-web-drop-in-react/dist/index.js:109,0][app.a2e9a6b7afa471d94d2b.js:116396,6]
As the error states, the issue is with the UglifyJs plugin, which I use in combination with WebPack.
This is a frustrating show-stopping problem. Unless fixed, I couldn’t complete a build.
Here’s what I had in my WebPack prod config:
new webpack.optimize.UglifyJsPlugin({ beautify: false, mangle: { screw_ie8: true, keep_fnames: true }, compress: { screw_ie8: true, warnings: false }, comments: false }),
This was only in my WebPack prod config. Therefore I didn’t notice any issue until trying to build for prod.
Now, in truth, I didn’t write the code / config above. I copy / pasted from somewhere else (I forget where) and as it just worked I didn’t pay much attention to it.
When it stopped working, I got sad, then got on to trying to fix it.
My Solution
There’s a bunch of suggested general solutions to this problem. A quick Google will turn up plenty of GitHub issues. Unfortunately none of them were specific to my exact error.
In my case, as best I understand it, the Braintree Web Drop In React should have compiled the dist.js file down to ES5, but is instead, in ES6. I concluded this based on this and this.
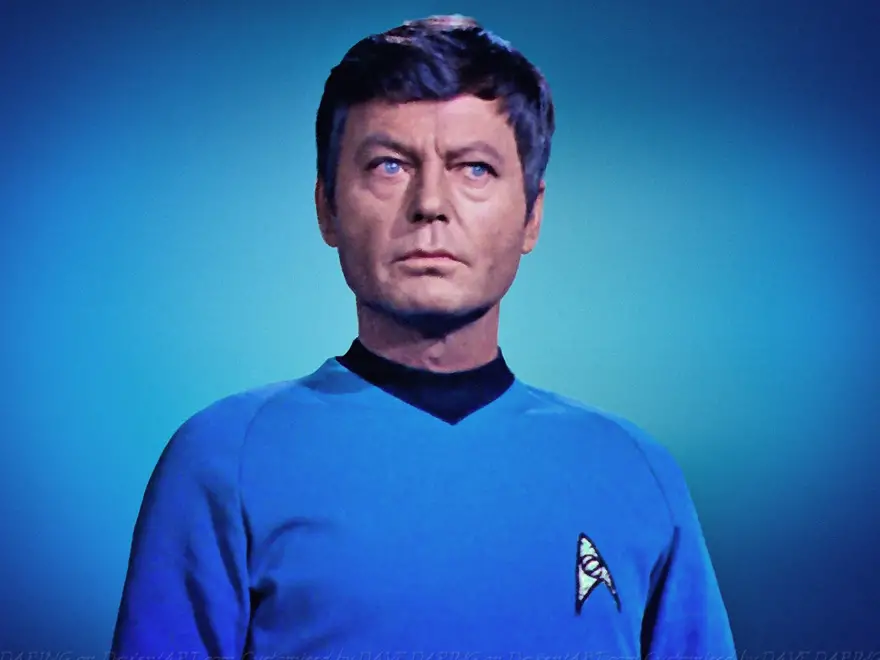
Of course, I may be wrong.
Fixing this wasn’t that hard. I just needed to read the docs.
yarn add --dev uglifyjs-webpack-plugin
For me, this added:
"uglifyjs-webpack-plugin": "^1.2.4",
To my devDependencies in package.json.
After which I updated my prod.js WebPack config as follows:
"use strict"; const webpack = require("webpack"); const UglifyJsPlugin = require('uglifyjs-webpack-plugin'); module.exports = function() { console.log("BUILDING PRODUCTION"); return webpackMerge(commonConfig(), { plugins: [ new UglifyJsPlugin({ test: /\.js($|\?)/i, sourceMap: true, uglifyOptions: { mangle: { keep_fnames: true, }, compress: { warnings: false, }, output: { beautify: false, }, }, }), }); };
I’ve removed everything unrelated to this specific problem.
After which I could get my build to work.